JavaScript Objects
Object Creation Functions, Inheritance, Properties, Methods, & Instantiation
The output from these buttons, but this took hours and hours to figure out. I have a much more solid grasp of JavaScript Objects now.
Dimensions of a Cube
I created a simple "Cube" object with properties of length, width, and height. For simplicity, I hard coded the values, but the values could come dynamically through various methods.
Object with Inheritance
I'm calling this a "layered" object, because one of the properties in this object is actually an object inherited from another instance. I wanted to create a function that would display the properties and values of an object dynamically. I used a for loop and a for/in loop to get this to display the way I wanted. Click the button below to see the magic.
FYI: I used console.log a lot for learning purposes. You may want to check it out to see what is there.
Properties
A property of an object is the name of the attribute. For example if I had a car object with the make of Honda, car.make would be the property.
Syntax is:
- objectName.property // car.make
- objectName["property"] // car["make"]
- objectName[expression] // x = "make"; car.x
You can think of an object property like a variable that "belongs" to the object. Property names can be dynamically determined. Dynamic property names needs to use square brackets [].
Show One property in an Object
Methods
Methods are when a function is a property of an object. Rather than having an object of Character {"first name": "Mama", "last name": "Bear","full name": "Mama Bear"}, we can make "full name" a function like this:
fullName: function () { return this.firstName + " " + this.lastName; }
This displays:
Inheritance & Instantiation
From MDN: All objects in JavaScript inherit from at least one other object. The object being inherited from is known as the prototype, and the inherited properties can be found in the prototype object of the constructor. In the screenshot below, the Instructor function is the prototype for future objects. Objects created as new instances of Instructor will inherit the properties of name, age, and gender from this function.
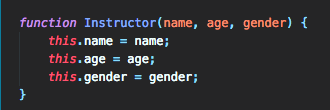
Constructor functions
The prototype is a constructor function. This allows you to create an instance of this object with the word new.
Object.creation
Object.create is very useful because it allows you to use the prototype object to create a new object without having to define a constructor function. You can see a screenshot of my code.
Check the console to see the out put of this function.
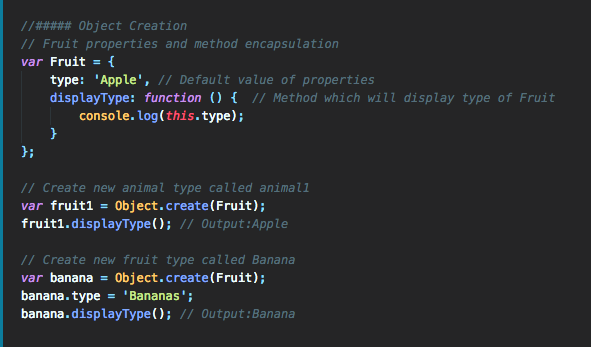
Aha! Moment
One of my big realizations while studying this subject is that much of the built-in functions we use in JavaScript are objects. For example DOM is the Document Object Model. Things like Number, Math, and Date are also objects. Those words perform functions when concatenated to other words because those words represent objects. Aha! This is important to know!
References: These instructions at MDN were very helpful.